Shuffle String leetcode solution in c++
You are given a string s
and an integer array indices
of the same length. The string s
will be shuffled such that the character at the ith
position moves to indices[i]
in the shuffled string.
Return the shuffled string.
Example 1:
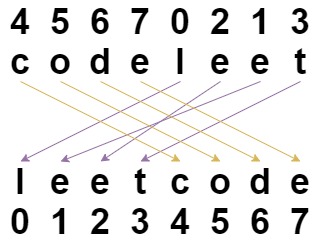
Input: s = "codeleet", indices
= [4,5,6,7,0,2,1,3]
Output: "leetcode"
Explanation: As shown, "codeleet" becomes "leetcode" after shuffling.
Example 2:
Input: s = "abc", indices
= [0,1,2]
Output: "abc"
Explanation: After shuffling, each character remains in its position.
Constraints:
s.length == indices.length == n
1 <= n <= 100
s
consists of only lowercase English letters.0 <= indices[i] < n
- All values of
indices
are unique.
solution<1>:
Runtime: 19 ms, faster than 20.85% of C++ online submissions for Shuffle String.
Memory Usage: 15.2 MB, less than 78.13% of C++ online submissions for Shuffle String.
- class Solution {
- public:
- string restoreString(string s, vector<int>& indices) {
- string ans=s;
- for (int i = 0; i < indices.size(); i++)
- {
- ans[indices[i]]=s[i];
- }
- return ans;
- }
- };
solution<2>:
Runtime: 25 ms, faster than 7.50% of C++ online submissions for Shuffle String.
Memory Usage: 15.3 MB, less than 42.34% of C++ online submissions for Shuffle String.
- class Solution {
public:
string restoreString(string s, vector<int>& indices) {
string ans;
for (int i = 0; i < indices.size(); i++)
{
for (int j = 0; j < indices.size(); j++)
{
if (i == indices[j])
{
ans += s[j];
}
}
}
return ans;
}
};
No comments:
Post a Comment